Introduction to Algorithm Analysis
Algorithm performance analysis is a fundamental step in the design of an algorithm. Given a computational problem, several solutions can be proposed, such as sorting algorithms like BubbleSort, MergeSort, and QuickSort. Understanding how these algorithms behave as the input size increases is essential for choosing the best solution in a given context.
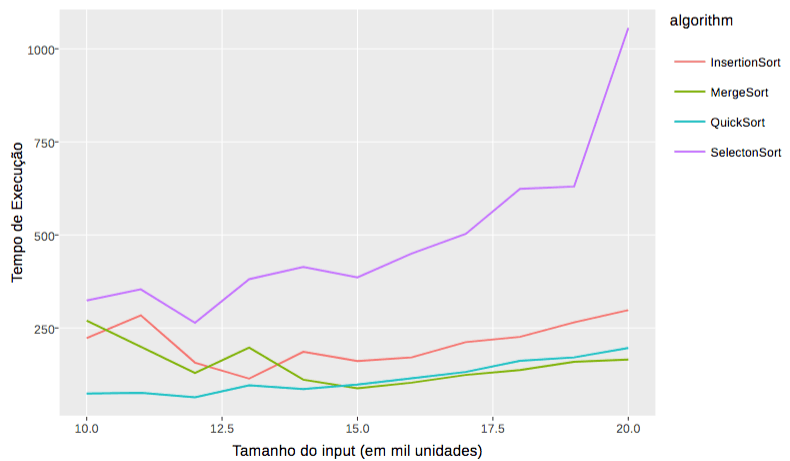
Empirical Analysis
An empirical approach analyzes the performance of an algorithm by setting up a controlled environment and measuring the execution time. The graph shows the comparison of different sorting algorithms as a function of the input size.
int multiplyRemainderByIntegerPart(int i, int j) {
int remainder = i % j;
int integerPart = i / j;
return remainder * integerPart;
}
The empirical approach is useful but has limitations, such as the high cost of implementation and dependence on the hardware. Therefore, asymptotic analysis is preferred, as it is independent of the hardware and covers a broader range of inputs.
Asymptotic Analysis
Asymptotic analysis focuses on the behavior of algorithms for large inputs. It is based on the assumption that the cost of primitive operations is constant (O(1)).
Example: Algorithm with Constant Cost
int multiplyRemainderByIntegerPart(int i, int j) {
int remainder = i % j;
int integerPart = i / j;
return remainder * integerPart;
}
In this example, the execution time is constant, regardless of the input size. For more complex algorithms, such as search or sorting, the execution time may vary depending on the number of iterations or elements in the array.
Worst Case
When there are loops or conditionals, analyzing the worst case is important to ensure the algorithm behaves as expected in extreme scenarios.
public static boolean contains(int[] v, int n) {
for (int i = 0; i < v.length; i++) {
if (v[i] == n)
return true;
}
return false;
}
For algorithms that involve iteration or nested loops, the execution time may be linear or quadratic, depending on the number of elements and the depth of the loops.
Asymptotic analysis helps us simplify these functions to understand the behavior of algorithms with large inputs, representing them through notations like O(n), O(log n), or O(n²), making it easier to compare and choose the best solution.
Algorithm analysis, both empirical and asymptotic, is essential to ensure the efficiency and performance of code, especially in scenarios involving large volumes of data or when performance is critical.